Making a VSTi Host - Part 4
- Tim Cave
- Jan 3, 2021
- 2 min read
If you are serious about making music then you will know about ASIO drivers.
In this blog I want to show:
How to play a wav file through an ASIO driver
How to play a VSTi plugin through ASIO
If you haven't got an ASIO driver then you can download ASIO4ALL from here.
The next step is to download the BASSASIO package from the bottom of the page here. Then copy across the header, lib and dll files to your project and set up like before.
Now add a button and listBox to your form and add this code to the header file:
const char* charstr = "";
int a;
BASS_ASIO_DEVICEINFO info;
for (a = 0; BASS_ASIO_GetDeviceInfo(a, &info); a++) {
charstr = info.name;
String^ clistr = gcnew String(charstr);
listBox1->Items->Add("Device number = " + a.ToString() + " with name " + clistr);
}
When you run this it will populate the list box with the drivers you have on your system and provide the driver device number, which you'll need for the next bit.
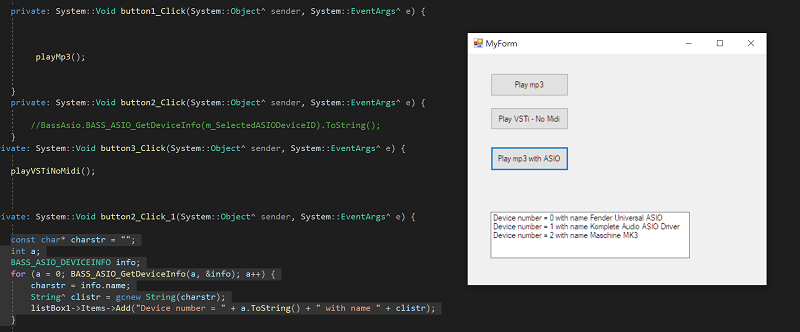
I want to use my Komplete Audio driver so I'll use device number 1.
Now add a new function below the above code and go over to the cpp code and add the following:
void playWithASIO() {
BASS_CHANNELINFO i;
BASS_Init(0, 44100, 0, 0, NULL);
DWORD chan = BASS_StreamCreateFile(FALSE, "c:\\temp\\tc.wav", 0, 0, BASS_SAMPLE_LOOP | BASS_STREAM_DECODE | BASS_SAMPLE_FLOAT);
//pick the ASIO driver you want to use
if (!BASS_ASIO_Init(1, BASS_ASIO_THREAD)) // initialize ASIO device
MessageBox::Show("Can't initialize ASIO device");
if (!BASS_ASIO_ChannelEnableBASS(FALSE, 0, chan, TRUE)) // enable ASIO channel(s)
MessageBox::Show("Can't enable ASIO channel(s)");
BASS_ChannelGetInfo(chan, &i);
if (i.chans == 1) BASS_ASIO_ChannelEnableMirror(1, FALSE, 0); // mirror mono channel to form stereo output
BASS_ASIO_SetRate(i.freq); // try to set the device rate to avoid resampling
BASS_ASIO_Start(0, 0); // start the device using default buffer/latency
MessageBox::Show("Playing");
BASS_ASIO_Free();
BASS_Free();
}
If all goes well you should be able to hear your file playing through your ASIO driver.
The final step today is to replace the channel stream with the VSTi stream. Remember to change the "flags" and use - BASS_SAMPLE_LOOP | BASS_STREAM_DECODE | BASS_SAMPLE_FLOAT
Add a new button and function and then use this code:
void playVSTiWithASIO() {
BASS_CHANNELINFO i;
BASS_Init(0, 44100, 0, 0, NULL);
//assign a VSTi plugin to the channel
DWORD chan = BASS_VST_ChannelCreate(44100, 2, "C:\\Program Files (x86)\\Vstplugins\\DSK The Grand.dll",
BASS_SAMPLE_LOOP | BASS_STREAM_DECODE | BASS_SAMPLE_FLOAT);
BASS_VST_INFO vstInfo; //vst info
//pick the ASIO driver you want to use
if (!BASS_ASIO_Init(1, BASS_ASIO_THREAD)) // initialize ASIO device
MessageBox::Show("Can't initialize ASIO device");
if (!BASS_ASIO_ChannelEnableBASS(FALSE, 0, chan, TRUE)) // enable ASIO channel(s)
MessageBox::Show("Can't enable ASIO channel(s)");
BASS_ChannelGetInfo(chan, &i);
if (i.chans == 1) BASS_ASIO_ChannelEnableMirror(1, FALSE, 0); // mirror mono channel to form stereo output
BASS_ASIO_SetRate(i.freq); // try to set the device rate to avoid resampling
BASS_ASIO_Start(0, 0); // start the device using default buffer/latency
//show editor
VSTiHostBlog::MyForm1 form;
if (BASS_VST_GetInfo(chan, &vstInfo) && vstInfo.hasEditor)
{
const char* charstr = "";
charstr = vstInfo.productName;
String^ clistr = gcnew String(charstr);
form.Text = clistr;
form.Width = vstInfo.editorWidth + 10;
form.Height = vstInfo.editorHeight + 40;
IntPtr iHwnd = form.Handle;
HWND hwnd = (HWND)iHwnd.ToInt32();
BASS_VST_EmbedEditor(chan, hwnd);
form.ShowDialog();
}
else {
MessageBox::Show("No editor");
}
form.Close();
BASS_ASIO_Free();
BASS_Free();
}
Hopefully you can now hear your VSTi playing through your ASIO driver.
Clearly the code needs sorting out a some point and I will come on to that soon!
This is just a demo after all... :>
Part 5 coming soon...
Comments