Making a VSTi Host - Part 2
- Tim Cave
- Jan 10, 2021
- 3 min read
Updated: Jan 14, 2021
I can remember the excitement of buying Type and Learn C with it's free Turbo C++ Compiler and putting "Hello World" on the screen. And then moving onto VC++, which you had to buy and arrived in big box with numerous floppy disks - it took all evening to load onto my 286 PC and about a week to work out how to get a button on form - heady days!
Anyway back to my VSTi Host in C++ and in this blog I will cover:
Get C++ Window form set up
Stream an mp3 file through a vst
Show the vst editor
Setting Up My Project
I'm using Visual Studio 2019 Community addition which is free to download.
At this point I almost hit a wall that stopped me in my tracks. I worked out that I wanted to create a C++/CLI Windows Forms app, which requires a bit of setting up.
I've subsequently downloaded the MS Step by Step book (free PDF) and understood that with C++/CLI you can use the .Net framework; you do need to get used to a slightly different syntax but it does at least look familiar.
//C++
System::Collections::Generic::List
System::Threading::Thread
//C#
System.Collections.Generic.List
System.Threading.Thread
To get a form loaded up in C++/CLI is not as straight forward as C# and you have to follow the steps here.
If all goes well you should have something like this:

Remember to include your MyForm.h include at the top of the code.
At this point you need to download BassVst dll and lib files along with the necessary header files. Follow the instructions here.
Back in VS2019 add a button to your form and double click it, this takes you through to header file. Now header files are designed to provide the information that will be needed in multiple files. Things like class declarations and function prototypes typically go in header files.
So I went for putting my first function here and then caling it in the cpp file.
I also needed to set up a GlobalFunctions header file.
So I did the following:
In MyForm.h I added a function.
private: System::Void button1_Click(System::Object^ sender, System::EventArgs^ e) {
void playMp3();
}
In GlobalFunctions.h I added.
void playMp3();
And in MyForm.cpp I added:
void playMp3() {
BASS_Init(-1, 44100, 0, 0, NULL);
// create a normal BASS stream
DWORD ch = BASS_StreamCreateFile(false, "c:\\temp\\test.mp3", 0, 0, 0);
// assign a VST plugin DLL to the channel -- the returned handle can be used
// with all BASS_VST_*() functions
DWORD dsp = BASS_VST_ChannelSetDSP(ch, "C:\\Program Files (x86)\\Vstplugins\\ambience.dll", 0, 0);
// that's all
BASS_ChannelPlay(ch, false);
MessageBox::Show("MP3 Playing");
// delete the stream - this will also delete the VST effect
BASS_StreamFree(ch);
}
I also add the headers at the top of the code:
Then copy over the bass.h and bassvst.h files to your source code folder and add them to your project under the header files by right clicking Header Files and choosing an Existing Item.
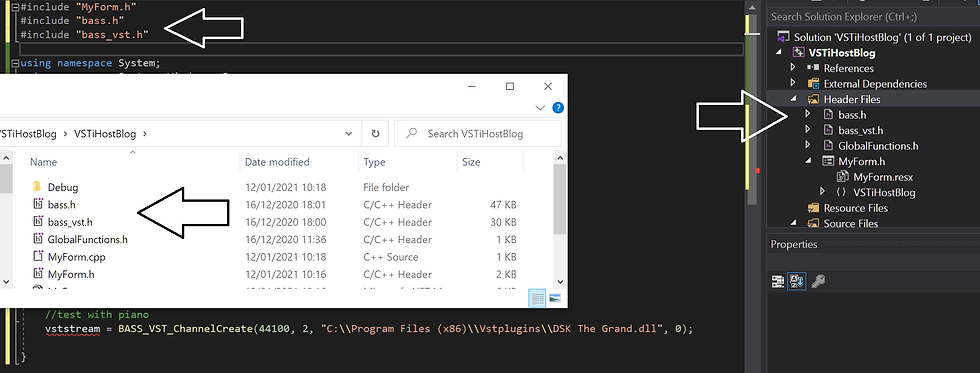
If you try compiling this you get an scary error about unresolved tokens - this is because the lib and dll files are not included.
The easy way to add the bass.dll is use the NuGet tool which you can load up under Tools -> NuGet Package Manager in Visual Studio. Search for Bass and include in your project.
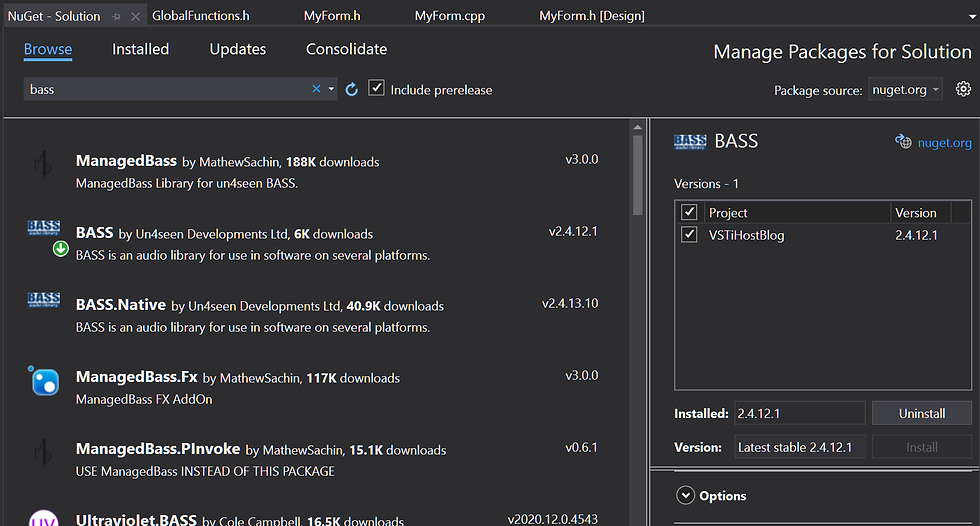
Unfortunately bass_vst doesn't have this facility so you have to copy the files to your debug directory - note you have to match the right files to your build. I'm using x86 bassvst.dll / bassvst.lib.
Go back to project properties and add the Additional Dependencies for the bass_vst.lib.

Now if you compile this now you may get an error saying cannot open the file bassvst.dll - if you get that go to file explorer and right click the file, select properties and check the Unblock checkbox at the bottom.
Now your project should compile and it should play your mp3 through the vst effect...time for drink?
Finally for this blog let's add an editor window to show the vst plugin!
Right click the project name at the top of the Solution Explorer and add a new form (under UI).
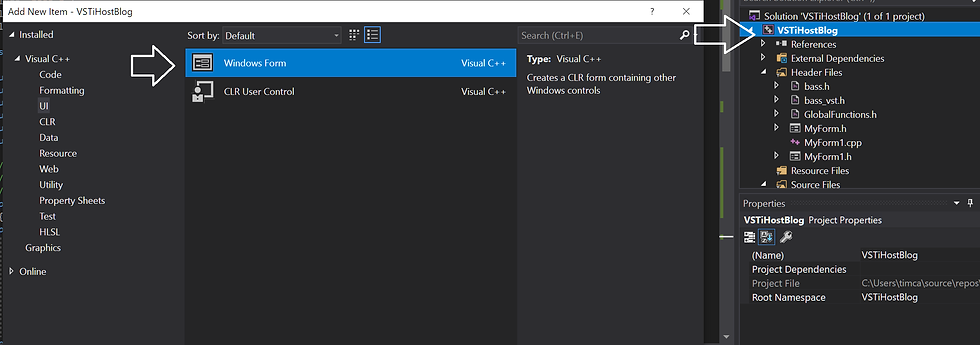
Then under the ChannelSetDSP line add this code:
BASS_VST_INFO vstInfo; //vst info
//show editor
VSTiHostBlog::MyForm1 form;
if (BASS_VST_GetInfo(dsp, &vstInfo) && vstInfo.hasEditor)
{
form.Width = vstInfo.editorWidth + 40;
form.Height = vstInfo.editorHeight + 40;
form.AutoScale;
IntPtr iHwnd = form.Handle;
HWND hwnd = (HWND)iHwnd.ToInt32();
BASS_VST_EmbedEditor(dsp, hwnd);
form.ShowDialog();
}
else {
MessageBox::Show("No editor");
}
form.Close();
The plugin form will open, if it has an editor, and then when you close it the mp3 will play.
Not ideal but it's a start and in the next blog I want to move on to showing the editor for a vsti and playing a midi file with that.
Go on to Part 3...
Commenti